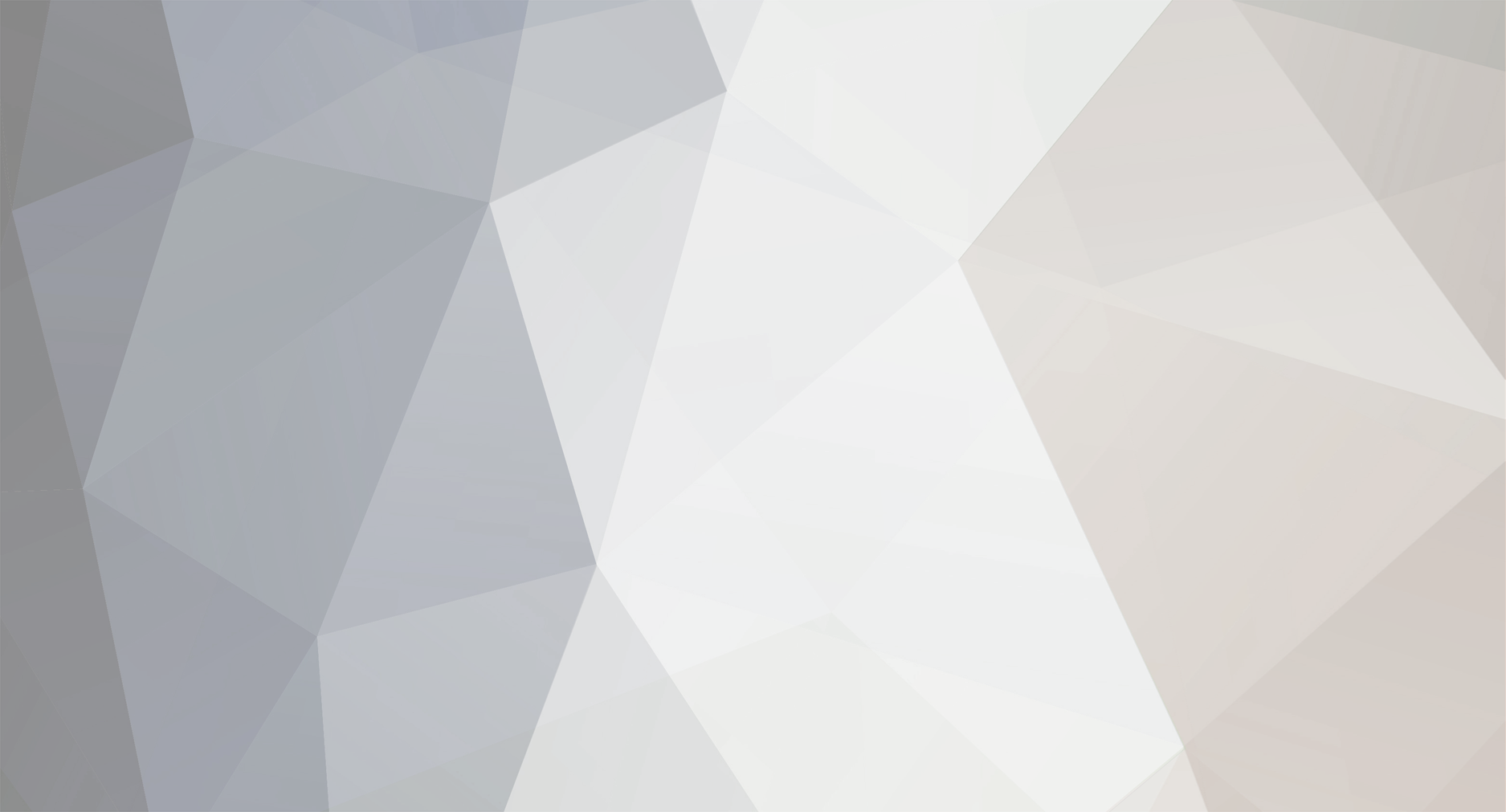
- Mariel
-
Content Count
80 -
Joined
-
Last visited
Posts posted by - Mariel
-
-
bump
-
/home/xxx/trunk/src/plugins/autopots.c:231: undefined reference to `sscanf_s'you need to include 'stdio.h'
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "../common/HPMi.h"
#include "../common/timer.h"
#include "../map/script.h"
#include "../map/pc.h"
#include "../map/map.h"
#include "../map/unit.h"
#include "../map/atcommand.h"
#include "../map/itemdb.h"
#include "../common/HPMDataCheck.h"/* should always be the last file included! (if you don't make it last, it'll intentionally break compile time) */
#define OPTION_AUTOPOTS 0x40000000
/*
3.1 Plugins Release [Mhalicot]
3.2 Update to Latest Revision [Mhalicot]
4.0 Added autohp, autosp command Rev. 137* [Mhalicot]
4.1 Fixed Compilation error. Rev. 145*** [Mhalicot]
-----------------------------------------------------
Documentation
@autopots
@autohp
@autosp // Change autopots/hp/sp timer by replacing +500 in milisec
timer->add(timer->gettick()+500,autoatpots_timer,sd->bl.id,0);
*/
HPExport struct hplugin_info pinfo = {
"autopots", // Plugin name
SERVER_TYPE_MAP, // Which server types this plugin works with?
"4.0", // Plugin version
HPM_VERSION, // HPM Version (don't change, macro is automatically updated)
};
struct autopots {
unsigned int hp_rate, sp_rate, hp_nameid, sp_nameid;
};
struct autopots autopots;
void autoatpots_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.hp_nameid = 0;
autopots.hp_rate = 0;
autopots.sp_nameid = 0;
autopots.sp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
void autoathp_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.hp_nameid = 0;
autopots.hp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
void autoatsp_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.sp_nameid = 0;
autopots.sp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
int autoatpots_timer(int tid, int64 tick, int id, intptr_t data)
{
struct map_session_data *sd=NULL;
struct item_data* item = NULL;
int index;
sd=map->id2sd(id);
if( sd == NULL )
return 0;
if(sd->sc.option & OPTION_AUTOPOTS)
{
unsigned int hp_rate = autopots.hp_rate;
unsigned int sp_rate = autopots.sp_rate;
unsigned int hp_nameid = autopots.hp_nameid;
unsigned int sp_nameid = autopots.sp_nameid;
if( ( !sp_rate && !hp_rate ) || pc_isdead(sd) )
{
if( !hp_rate )
{
clif->message(sd->fd, "Auto-SP : OFF_");
autoatsp_clean(sd);
return 0;
}else
if( !sp_rate )
{
clif->message(sd->fd, "Auto-HP : OFF_");
autoathp_clean(sd);
return 0;
}
else{
clif->message(sd->fd, "Auto-pots : OFF_");
autoatpots_clean(sd);
return 0;
}
}
if( ( sd->battle_status.hp*100/sd->battle_status.max_hp ) < hp_rate && hp_nameid && hp_rate )
{
ARR_FIND(0, MAX_INVENTORY, index, sd->status.inventory[index].nameid == hp_nameid);
if( sd->status.inventory[index].nameid == hp_nameid )
pc->useitem(sd,index);
}
if( ( sd->battle_status.sp*100/sd->battle_status.max_sp ) < sp_rate && sp_nameid && sp_rate )
{
ARR_FIND(0, MAX_INVENTORY, index, sd->status.inventory[index].nameid == sp_nameid);
if( sd->status.inventory[index].nameid == sp_nameid )
pc->useitem(sd,index);
}
timer->add(timer->gettick()+500,autoatpots_timer,sd->bl.id,0);
}
return 0;
}
ACMD(autopots)
{
int hp_rate=0, hp_nameid=0, sp_rate=0, sp_nameid=0;
if( !sd ) return 0;
if ( !message || !*message || (
sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) < 4) ||
( hp_rate < 0 || hp_rate > 99 ) ||
( sp_rate < 0 || sp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) < 4 &&
sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) > 0)
{
clif->message(fd, "@autopots ");
return false;
}
clif->message(fd, "Auto-pots : OFF");
autoatpots_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoatpots_clean(sd);
}
if( hp_rate == 0 ) hp_nameid = 0;
if( sp_rate == 0 ) sp_nameid = 0;
if( hp_nameid == 0 ) hp_rate = 0;
if( sp_nameid == 0 ) sp_rate = 0;
if( itemdb->exists(hp_nameid) == NULL && hp_nameid )
{
hp_nameid = 0;
hp_rate = 0;
clif->message(fd, "Auto-pots : Invalid item for HP");
}
if( itemdb->exists(sp_nameid) == NULL && sp_nameid )
{
sp_nameid = 0;
sp_rate = 0;
clif->message(fd, "Auto-pots : Invalid item for SP");
return true;
}
clif->message(fd, "Auto-pots : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.hp_nameid = hp_nameid;
autopots.hp_rate = hp_rate;
autopots.sp_nameid = sp_nameid;
autopots.sp_rate = sp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
ACMD(autohp)
{
int hp_rate=0, hp_nameid=0;
if( !sd ) return 0;
if (!message || !*message || (
sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) < 2) ||
( hp_rate < 0 || hp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) < 2 &&
sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) > 0)
{
clif->message(fd, "@autohp ");
return true;
}
clif->message(fd, "Auto-HP : OFF");
autoathp_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoathp_clean(sd);
}
if( hp_rate == 0 ) hp_nameid = 0;
if( hp_nameid == 0 ) hp_rate = 0;
if( itemdb->exists(hp_nameid) == NULL && hp_nameid )
{
hp_nameid = 0;
hp_rate = 0;
clif->message(fd, "Auto-HP : Invalid item for HP");
return true;
}
clif->message(fd, "Auto-HP : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.hp_nameid = hp_nameid;
autopots.hp_rate = hp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
ACMD(autosp)
{
int sp_rate=0, sp_nameid=0;
if( !sd ) return 0;
if (!message || !*message || (
sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) < 2) ||
( sp_rate < 0 || sp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) < 2 &&
sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) > 0)
{
clif->message(fd, "@autosp ");
return true;
}
clif->message(fd, "Auto-SP : OFF");
autoatsp_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoatsp_clean(sd);
}
if( sp_rate == 0 ) sp_nameid = 0;
if( sp_nameid == 0 ) sp_rate = 0;
if( itemdb->exists(sp_nameid) == NULL && sp_nameid )
{
sp_nameid = 0;
sp_rate = 0;
clif->message(fd, "Auto-SP : Invalid item for SP");
return true;
}
clif->message(fd, "Auto-SP : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.sp_nameid = sp_nameid;
autopots.sp_rate = sp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
/* Server Startup */
HPExport void plugin_init (void)
{
clif = GET_SYMBOL("clif");
script = GET_SYMBOL("script");
pc = GET_SYMBOL("pc");
atcommand = GET_SYMBOL("atcommand");
map = GET_SYMBOL("map");
unit = GET_SYMBOL("unit");
timer = GET_SYMBOL("timer");
itemdb = GET_SYMBOL("itemdb");
addAtcommand("autopots",autopots);//link our '@autopots' command
addAtcommand("autohp",autohp);//link our '@autohp' command
addAtcommand("autosp",autosp);//link our '@autosp' command
}
make[1]: Entering directory `/home/xxx/trunk/src/plugins'
CC autopots.c
autopots.c: In function 'autoatpots_timer':
autopots.c:79: warning: unused variable 'item'
autopots.c: In function 'atcommand_autopots':
autopots.c:133: warning: implicit declaration of function 'sscanf_s'
autopots.c: In function 'autoatpots_timer':
autopots.c:114: warning: array subscript is above array bounds
autopots.c:120: warning: array subscript is above array bounds
/tmp/cceKjLNC.o: In function `atcommand_autosp':
/home/xxx/trunk/src/plugins/autopots.c:231: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:232: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:228: undefined reference to `sscanf_s'
/tmp/cceKjLNC.o: In function `atcommand_autohp':
/home/xxx/trunk/src/plugins/autopots.c:189: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:190: undefined reference to `sscanf_s'
/tmp/cceKjLNC.o:/home/xxx/trunk/src/plugins/autopots.c:186: more undefined references to `sscanf_s' follow
collect2: ld returned 1 exit status
make[1]: *** [../../plugins/autopots.so] Error 1
make[1]: Leaving directory `/home/xxx/trunk/src/plugins'
make: *** [plugin.autopots] Error 2
still no luck
using revision 16772
-
+1. Cheers to those who can't afford harmony. Lol.
-
bump
-
Guy,..Dont just appreciate donate him coffee for this project as its NOT a small or easy project and releasing it free is something HUGEE thing.
I always feel why is he doing it for free..
anyways
All the Best Dastgir and keep it up for your Hardwork and Efforts.
I think he just do it because he likes it and it makes him happy.
Thank you Dastgir!
-
-
bump
-
why you need to paste the autopots.so? when you compile autopots.so it will automatically generate autopots.so...
Make sure you know this guide
Oh it's a typo. I mean autopots.c
Is it errors or Warning? if, warning only you can ignore it.. check if it successfully build..
Error in building the plugin. Save as above, the only difference is that trunk folder is replaced by Hercules folder.
-
why you need to paste the autopots.so? when you compile autopots.so it will automatically generate autopots.so...
Make sure you know this guide
Oh it's a typo. I mean autopots.c
-
I dont have autopots,so because im using Windows, so i compile it using MSVS.
I don't know what to do. Thanks anyway!
Update first your hercules and let me know if still have problem.
I already did a fresh install of hercules using 'git clone https[color=rgb(102,102,0);:[/color]//github.com/HerculesWS/Hercules.git ~/Hercules']
then git pull
then ./configure
then make clean
pasted in src/plugins autopots.so
edited makefile.in
then make plugins
still the same errors
-
I dont have autopots,so because im using Windows, so i compile it using MSVS.
I don't know what to do. Thanks anyway!
-
can I ask? why there is an empty #include in there? is it typo error? can you remove it in your code and recompile @autopots check if still have error?
if theres error try to use autotpots
still the same
weird. sorry can't help its working my latest SVN.. is your server updated?
I'll try pulling a new hercules and try make plugins. If you upload autopots.so, will my server load that plugin ?
-
can I ask? why there is an empty #include in there? is it typo error? can you remove it in your code and recompile @autopots check if still have error?
if theres error try to use autotpots
still the same
-
can you share me your codes? i really can't reproduce your error
#include
#include
#include
#include "../common/HPMi.h"
#include "../common/timer.h"
#include "../map/script.h"
#include "../map/pc.h"
#include "../map/map.h"
#include "../map/unit.h"
#include "../map/atcommand.h"
#include "../map/itemdb.h"
#include "../common/HPMDataCheck.h"/* should always be the last file included! (if you don't make it last, it'll intentionally break compile time) */
#define OPTION_AUTOPOTS 0x40000000
/*
3.1 Plugins Release [Mhalicot]
3.2 Update to Latest Revision [Mhalicot]
4.0 Added autohp, autosp command Rev. 137* [Mhalicot]
4.1 Fixed Compilation error. Rev. 145*** [Mhalicot]
-----------------------------------------------------
Documentation
@autopots
@autohp
@autosp // Change autopots/hp/sp timer by replacing +500 in milisec
timer->add(timer->gettick()+500,autoatpots_timer,sd->bl.id,0);
*/
HPExport struct hplugin_info pinfo = {
"autopots", // Plugin name
SERVER_TYPE_MAP, // Which server types this plugin works with?
"4.0", // Plugin version
HPM_VERSION, // HPM Version (don't change, macro is automatically updated)
};
struct autopots {
unsigned int hp_rate, sp_rate, hp_nameid, sp_nameid;
};
struct autopots autopots;
void autoatpots_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.hp_nameid = 0;
autopots.hp_rate = 0;
autopots.sp_nameid = 0;
autopots.sp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
void autoathp_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.hp_nameid = 0;
autopots.hp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
void autoatsp_clean(struct map_session_data *sd)
{
if( sd )
{
sd->sc.option &= ~OPTION_AUTOPOTS;
autopots.sp_nameid = 0;
autopots.sp_rate = 0;
clif->changeoption(&sd->bl);
}
return;
}
int autoatpots_timer(int tid, int64 tick, int id, intptr_t data)
{
struct map_session_data *sd=NULL;
struct item_data* item = NULL;
int index;
sd=map->id2sd(id);
if( sd == NULL )
return 0;
if(sd->sc.option & OPTION_AUTOPOTS)
{
unsigned int hp_rate = autopots.hp_rate;
unsigned int sp_rate = autopots.sp_rate;
unsigned int hp_nameid = autopots.hp_nameid;
unsigned int sp_nameid = autopots.sp_nameid;
if( ( !sp_rate && !hp_rate ) || pc_isdead(sd) )
{
if( !hp_rate )
{
clif->message(sd->fd, "Auto-SP : OFF_");
autoatsp_clean(sd);
return 0;
}else
if( !sp_rate )
{
clif->message(sd->fd, "Auto-HP : OFF_");
autoathp_clean(sd);
return 0;
}
else{
clif->message(sd->fd, "Auto-pots : OFF_");
autoatpots_clean(sd);
return 0;
}
}
if( ( sd->battle_status.hp*100/sd->battle_status.max_hp ) < hp_rate && hp_nameid && hp_rate )
{
ARR_FIND(0, MAX_INVENTORY, index, sd->status.inventory[index].nameid == hp_nameid);
if( sd->status.inventory[index].nameid == hp_nameid )
pc->useitem(sd,index);
}
if( ( sd->battle_status.sp*100/sd->battle_status.max_sp ) < sp_rate && sp_nameid && sp_rate )
{
ARR_FIND(0, MAX_INVENTORY, index, sd->status.inventory[index].nameid == sp_nameid);
if( sd->status.inventory[index].nameid == sp_nameid )
pc->useitem(sd,index);
}
timer->add(timer->gettick()+500,autoatpots_timer,sd->bl.id,0);
}
return 0;
}
ACMD(autopots)
{
int hp_rate=0, hp_nameid=0, sp_rate=0, sp_nameid=0;
if( !sd ) return 0;
if ( !message || !*message || (
sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) < 4) ||
( hp_rate < 0 || hp_rate > 99 ) ||
( sp_rate < 0 || sp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) < 4 &&
sscanf_s(message, "%d %d %d %d ", &hp_rate, &hp_nameid, &sp_rate, &sp_nameid) > 0)
{
clif->message(fd, "@autopots ");
return false;
}
clif->message(fd, "Auto-pots : OFF");
autoatpots_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoatpots_clean(sd);
}
if( hp_rate == 0 ) hp_nameid = 0;
if( sp_rate == 0 ) sp_nameid = 0;
if( hp_nameid == 0 ) hp_rate = 0;
if( sp_nameid == 0 ) sp_rate = 0;
if( itemdb->exists(hp_nameid) == NULL && hp_nameid )
{
hp_nameid = 0;
hp_rate = 0;
clif->message(fd, "Auto-pots : Invalid item for HP");
}
if( itemdb->exists(sp_nameid) == NULL && sp_nameid )
{
sp_nameid = 0;
sp_rate = 0;
clif->message(fd, "Auto-pots : Invalid item for SP");
return true;
}
clif->message(fd, "Auto-pots : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.hp_nameid = hp_nameid;
autopots.hp_rate = hp_rate;
autopots.sp_nameid = sp_nameid;
autopots.sp_rate = sp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
ACMD(autohp)
{
int hp_rate=0, hp_nameid=0;
if( !sd ) return 0;
if (!message || !*message || (
sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) < 2) ||
( hp_rate < 0 || hp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) < 2 &&
sscanf_s(message, "%d %d ", &hp_rate, &hp_nameid) > 0)
{
clif->message(fd, "@autohp ");
return true;
}
clif->message(fd, "Auto-HP : OFF");
autoathp_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoathp_clean(sd);
}
if( hp_rate == 0 ) hp_nameid = 0;
if( hp_nameid == 0 ) hp_rate = 0;
if( itemdb->exists(hp_nameid) == NULL && hp_nameid )
{
hp_nameid = 0;
hp_rate = 0;
clif->message(fd, "Auto-HP : Invalid item for HP");
return true;
}
clif->message(fd, "Auto-HP : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.hp_nameid = hp_nameid;
autopots.hp_rate = hp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
ACMD(autosp)
{
int sp_rate=0, sp_nameid=0;
if( !sd ) return 0;
if (!message || !*message || (
sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) < 2) ||
( sp_rate < 0 || sp_rate > 99 ) )
{
if ( sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) < 2 &&
sscanf_s(message, "%d %d ", &sp_rate, &sp_nameid) > 0)
{
clif->message(fd, "@autosp ");
return true;
}
clif->message(fd, "Auto-SP : OFF");
autoatsp_clean(sd);
return true;
}
if (sd->sc.option & OPTION_AUTOPOTS)
{
autoatsp_clean(sd);
}
if( sp_rate == 0 ) sp_nameid = 0;
if( sp_nameid == 0 ) sp_rate = 0;
if( itemdb->exists(sp_nameid) == NULL && sp_nameid )
{
sp_nameid = 0;
sp_rate = 0;
clif->message(fd, "Auto-SP : Invalid item for SP");
return true;
}
clif->message(fd, "Auto-SP : ON");
sd->sc.option |= OPTION_AUTOPOTS;
autopots.sp_nameid = sp_nameid;
autopots.sp_rate = sp_rate;
timer->add(timer->gettick()+200,autoatpots_timer,sd->bl.id,0);
clif->changeoption(&sd->bl);
return true;
}
/* Server Startup */
HPExport void plugin_init (void)
{
clif = GET_SYMBOL("clif");
script = GET_SYMBOL("script");
pc = GET_SYMBOL("pc");
atcommand = GET_SYMBOL("atcommand");
map = GET_SYMBOL("map");
unit = GET_SYMBOL("unit");
timer = GET_SYMBOL("timer");
itemdb = GET_SYMBOL("itemdb");
addAtcommand("autopots",autopots);//link our '@autopots' command
addAtcommand("autohp",autohp);//link our '@autohp' command
addAtcommand("autosp",autosp);//link our '@autosp' command
}
More power to you Mhalicot. :> Thanks in advance.
-
CC autopots.c
autopots.c: In function 'autoatpots_timer':
autopots.c:79: warning: unused variable 'item'
autopots.c: In function 'atcommand_autopots':
autopots.c:133: warning: implicit declaration of function 'sscanf_s'
autopots.c: In function 'autoatpots_timer':
autopots.c:114: warning: array subscript is above array bounds
autopots.c:120: warning: array subscript is above array bounds
/tmp/ccqykVpt.o: In function `atcommand_autosp':
/home/xxx/trunk/src/plugins/autopots.c:231: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:232: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:228: undefined reference to `sscanf_s'
/tmp/ccqykVpt.o: In function `atcommand_autohp':
/home/xxx/trunk/src/plugins/autopots.c:189: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:190: undefined reference to `sscanf_s'
/tmp/ccqykVpt.o:/home/xxx/trunk/src/plugins/autopots.c:186: more undefined references to `sscanf_s' follow
collect2: ld returned 1 exit status
make[1]: *** [../../plugins/autopots.so] Error 1
make[1]: Leaving directory `/home/xxx/trunk/src/plugins'
make: *** [plugins] Error 2Any one who knows how to fix this ? Thanks in advance.
Edit : I tried using the fresh latest revision, and make plugins. Still the same error.
-
I just saw this in your signature. Omg. <3 Amazing!
-
inter-server.conf should look like this
// Hercules InterServer (settings shared/used by more than 1 server) configuration.
// Options for both versions
// Log Inter Connections, etc.?
log_inter: 1
// Inter Log Filename
inter_log_filename: log/inter.log
// Level range for sharing within a party
party_share_level: 15
// SQL version options only
// You can specify the codepage to use in your mySQL tables here.
// (Note that this feature requires MySQL 4.1+)
//default_codepage:
// For IPs, ideally under linux, you want to use localhost instead of 127.0.0.1
// Under windows, you want to use 127.0.0.1. If you see a message like
// "Can't connect to local MySQL server through socket '/tmp/mysql.sock' (2)"
// and you have localhost, switch it to 127.0.0.1
// Global SQL settings
// overridden by local settings when the hostname is defined there
// (currently only the login-server reads/obeys these settings)
sql.db_hostname: 127.0.0.1
sql.db_port: 3306
sql.db_username: rootsql.db_password: ragnarok
sql.db_database: hercules
sql.codepage:BattleGrounds reacted to this -
Can you download notepad++ and repost the pictures here.
-
//====================================================
//= _ _ _
//= | | | | | |
//= | |_| | ___ _ __ ___ _ _| | ___ ___
//= | _ |/ _ '__/ __| | | | |/ _ / __|
//= | | | | __/ | | (__| |_| | | __/__
//= _| |_/___|_| ___|__,_|_|___||___/
//=
//= http://herc.ws/board/
//====================================================
//= Hercules Map Zone Database [ind/Hercules]
//================ More Information ==================
//= http://herc.ws/board/topic/302-introducing-hercules-map-zone-database/
//= (TODO: replace with wiki link and detail the wiki page in a decent format ^)
//====================================================
//================ Description =======================
//= A unlimited number of zones may be created, a zone
//= may be used to create a set of disabled items, disabled skills
//= and mapflags to be used by as many maps as one chooses.
//= Maps can be linked to a specific zone through the zone mapflag
//= 'mapflagzone'.
//====================================================
//= Available types for 'disabled_skills':
//= PLAYER, HOMUN, MERCENARY, MONSTER, PET, ELEMENTAL, MOB_BOSS, CLONE, ALL and NONE
//====================================================
zones: (
{
/* All zone is a dynamic (very special) zone that is forcebly inherited by ALL maps automatically */
name: "All" /* changing this name requires MAP_ZONE_ALL_NAME to also be changed in src/map/map.h file */
disabled_skills: {
//both examples below disable napalm beat (id 11) to players
//MG_NAPALMBEAT: "PLAYER"
//ID11: "PLAYER"
}
disabled_items: {
//Both examples below disable apple (id 501)
//Apple: true
//ID501: true
}
mapflags: (
)
/* "command:min-group-lv-to-override" e.g. "heal: 70" */
disabled_commands: {
//Example Below makes @heal be used in maps within this zone only by those group lv 70 and above
//heal: 70
}
skill_damage_cap: {
//Exemple Below caps firebolt damage in maps within this zone to a maximum 50 damage,
// (depends on HMAP_ZONE_DAMAGE_CAP_TYPE in src/config/core.h)
// when cast vs players and monsters.
//MG_COLDBOLT: (50,"PLAYER | MONSTER")
}
},
{
/* Normal zone is applied to all maps that are not pkable (where players cant fight each other) */
/* However, it wont be applied to maps with its own zones (specified through mapflag) */
name: "Normal" /* changing this name requires MAP_ZONE_NORMAL_NAME to also be changed in src/map/map.h file */
disabled_skills: {
WM_LULLABY_DEEPSLEEP: "PLAYER"
WM_SIRCLEOFNATURE: "PLAYER"
WM_SATURDAY_NIGHT_FEVER: "PLAYER"
SO_ARRULLO: "PLAYER"
CG_HERMODE: "PLAYER"
}
disabled_items: {
}
mapflags: (
)
},
{
/* PvP zone is applied to all maps with a pvp mapflag */
name: "PvP" /* changing this name requires MAP_ZONE_PVP_NAME to also be changed in src/map/map.h file */
disabled_skills: {
BS_GREED: "PLAYER"
CG_HERMODE: "PLAYER"
}
disabled_items: {
Greed_Scroll: true
Wing_Of_Fly: true
Wing_Of_Butterfly: true
}
mapflags: (
"nocashshop",
)
},
{
/* PK Mode zone is only used when server is on pk_mode (battle.conf),
it applies to all pvp maps that don't have their own zone */
name: "PK Mode" /* changing this name requires MAP_ZONE_PK_NAME to also be changed in src/map/map.h file */
disabled_skills: {
}
disabled_items: {
}
/* PK Mode Damage Reductions */
/* - weapon_damage_rate -40% */
/* - magic_damage_rate -40% */
/* - misc_damage_rate -40% */
/* - long_damage_rate -30% */
/* - short_damage_rate -20% */
mapflags: (
"weapon_damage_rate 60",
"magic_damage_rate 60",
"misc_damage_rate 60",
"long_damage_rate 70",
"short_damage_rate 80",
)
},
{
/* GvG zone is applied to all maps with a gvg mapflag */
name: "GvG" /* changing this name requires MAP_ZONE_GVG_NAME to also be changed in src/map/map.h file */
disabled_skills: {
AL_TELEPORT: "PLAYER"
AL_WARP: "PLAYER"
WZ_ICEWALL: "PLAYER"
TF_BACKSLIDING: "PLAYER"
RG_INTIMIDATE: "PLAYER"
WE_CALLPARTNER: "PLAYER"
HP_ASSUMPTIO: "PLAYER"
HP_BASILICA: "PLAYER"
CG_MOONLIT: "PLAYER"
WE_CALLPARENT: "PLAYER"
WE_CALLBABY: "PLAYER"
CR_CULTIVATION: "PLAYER"
NJ_KIRIKAGE: "PLAYER"
CASH_ASSUMPTIO: "PLAYER"
BS_GREED: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
}
disabled_items: {
Assumptio_5_Scroll: true
Greed_Scroll: true
Pty_Assumptio_Scroll: true
Amon_Ra_Card: true
B_Eremes_Card: true
Atroce_Card: true
Bacsojin_Card: true
Baphomet_Card: true
Berzebub_Card: true
Dark_Lord_Card: true
Detale_Card: true
Doppelganger_Card: true
Dracula_Card: true
Drake_Card: true
Eddga_Card: true
Dark_Snake_Lord_Card: true
Fallen_Bishop_Card: true
Garm_Card: true
Gloom_Under_Night_Card: true
Golden_Bug_Card: true
B_Magaleta_Card: true
B_Katrinn_Card: true
B_Harword_Card: true
B_Seyren_Card: true
B_Shecil_Card: true
Ifrit_Card: true
Incant_Samurai_Card: true
Kiel_Card: true
Knight_Windstorm_Card: true
Ktullanux_Card: true
Lady_Tanee_Card: true
Lord_Of_Death_Card: true
Maya_Card: true
Mistress_Card: true
Moonlight_Flower_Card:
Orc_Hero_Card: true
Orc_Load_Card: true
Osiris_Card: true
Phreeoni_Card: true
Pharaoh_Card: true
Rsx_0806_Card: true
Randgris_Card: true
Tao_Gunka_Card: true
Thanatos_Card: true
Apocalips_H_Card: true
B_Ygnizem_Card: true
Ghostring_Card: true
Angeling_Card: true
}
disabled_commands: {
//Example Below makes @heal be used in maps within this zone only by those group lv 70 and above
//heal: 70
storage: 70
}
/* 5 second duration increase on GvG */
/* knockback disabled */
/* GvG Mode Damage Reductions */
/* - weapon_damage_rate -40% */
/* - magic_damage_rate -40% */
/* - misc_damage_rate -40% */
/* - long_damage_rate -20% */
/* - short_damage_rate -20% */
mapflags: (
"invincible_time_inc 5000",
"noknockback",
"weapon_damage_rate 60",
"magic_damage_rate 60",
"misc_damage_rate 60",
"long_damage_rate 80",
"short_damage_rate 80",
"nocashshop",
"gvg_noparty",
)
},
{
/* Battlegrounds zone is applied to all maps with a battlegrounds mapflag */
name: "Battlegrounds" /* changing this name requires MAP_ZONE_BG_NAME to also be changed in src/map/map.h file */
disabled_skills: {
AL_TELEPORT: "PLAYER"
AL_WARP: "PLAYER"
WZ_ICEWALL: "PLAYER"
TF_BACKSLIDING: "PLAYER"
RG_INTIMIDATE: "PLAYER"
MO_BODYRELOCATION: "PLAYER"
WE_CALLPARTNER: "PLAYER"
HP_ASSUMPTIO: "PLAYER"
HP_BASILICA: "PLAYER"
CG_MOONLIT: "PLAYER"
WE_CALLPARENT: "PLAYER"
WE_CALLBABY: "PLAYER"
CR_CULTIVATION: "PLAYER"
TK_RUN: "PLAYER"
TK_HIGHJUMP: "PLAYER"
SG_FEEL: "PLAYER"
SG_SUN_WARM: "PLAYER"
SG_MOON_WARM: "PLAYER"
SG_STAR_WARM: "PLAYER"
SG_SUN_COMFORT: "PLAYER"
SG_MOON_COMFORT: "PLAYER"
SG_STAR_COMFORT: "PLAYER"
SG_HATE: "PLAYER"
SG_SUN_ANGER: "PLAYER"
SG_MOON_ANGER: "PLAYER"
SG_STAR_ANGER: "PLAYER"
SG_SUN_BLESS: "PLAYER"
SG_MOON_BLESS: "PLAYER"
SG_STAR_BLESS: "PLAYER"
NJ_KIRIKAGE: "PLAYER"
CASH_ASSUMPTIO: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
}
disabled_items: {
Assumptio_5_Scroll: true
Pty_Assumptio_Scroll: true
}
/* knockback disabled */
/* Battlegrounds Damage Reductions */
/* - weapon_damage_rate -30% */
/* - magic_damage_rate -30% */
/* - misc_damage_rate -30% */
/* - long_damage_rate -25% */
/* - short_damage_rate -25% */
mapflags: (
"noknockback",
"weapon_damage_rate 70",
"magic_damage_rate 70",
"misc_damage_rate 70",
"long_damage_rate 75",
"short_damage_rate 75",
)
},
{
name: "Aldebaran Turbo Track"
disabled_skills: {
SM_ENDURE: "PLAYER"
AL_TELEPORT: "PLAYER"
AL_WARP: "PLAYER"
AL_CURE: "PLAYER"
TF_HIDING: "PLAYER"
WZ_ICEWALL: "PLAYER"
AS_CLOAKING: "PLAYER"
RG_INTIMIDATE: "PLAYER"
MO_BODYRELOCATION: "PLAYER"
LK_CONCENTRATION: "PLAYER"
LK_BERSERK: "PLAYER"
HP_BASILICA: "PLAYER"
WS_CARTBOOST: "PLAYER"
ST_CHASEWALK: "PLAYER"
CG_MOONLIT: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
GN_CARTBOOST: "PLAYER"
}
disabled_items: {
Wing_Of_Fly: true
Anodyne: true
Green_Potion: true
Panacea: true
}
},
{
name: "Jail"
disabled_skills: {
TK_JUMPKICK: "PLAYER"
TK_HIGHJUMP: "PLAYER"
}
disabled_items: {
Wing_Of_Fly: true
Wing_Of_Butterfly: true
Giant_Fly_Wing: true
WOB_Rune: true
WOB_Schwaltz: true
WOB_Rachel: true
WOB_Local: true
}
},
{
name: "Izlude Battle Arena"
disabled_skills: {
RG_INTIMIDATE: "PLAYER"
AL_TELEPORT: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
}
disabled_items: {
Wing_Of_Fly: true
}
},
{
name: "GvG2"
inherit: ( "GvG" ) /* will import all gvg has */
disabled_skills: {
TK_HIGHJUMP: "PLAYER"
SA_ABRACADABRA: "PLAYER"
}
},
{
name: "Sealed Shrine"
disabled_skills: {
MG_SAFETYWALL: "PLAYER"
AL_TELEPORT: "PLAYER | MONSTER | HOMUN | MERCENARY"
RG_INTIMIDATE: "PLAYER | MONSTER"
HP_ASSUMPTIO: "PLAYER"
CASH_ASSUMPTIO: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
}
},
{
name: "Memorial Dungeon" /* ETower, Orc's Memory, Nidhoggur's Nest, etc */
disabled_skills: {
AL_TELEPORT: "PLAYER | MONSTER | HOMUN | MERCENARY"
WZ_ICEWALL: "PLAYER"
RG_INTIMIDATE: "PLAYER | MONSTER"
PF_SPIDERWEB: "PLAYER"
NPC_EXPULSION: "PLAYER"
SC_FATALMENACE: "PLAYER"
SC_DIMENSIONDOOR: "PLAYER"
}
},
{
name: "Towns"
disabled_skills: {
AM_CANNIBALIZE: "PLAYER"
AM_SPHEREMINE: "PLAYER"
CR_CULTIVATION: "PLAYER"
BS_GREED: "PLAYER"
SC_MANHOLE: "PLAYER"
WM_POEMOFNETHERWORLD: "PLAYER"
GN_WALLOFTHORN: "PLAYER"
}
disabled_items: {
Greed_Scroll: true
}
}
)Knockback and @storage is still enabled in gvg maps. Also mvp cards are also enabled.
Solved. Line 186.
-
Hercules Development Team presents
_ _ _
| | | | | |
| |_| | ___ _ __ ___ _ _| | ___ ___
| _ |/ _ '__/ __| | | | |/ _ / __|
| | | | __/ | | (__| |_| | | __/__
_| |_/___|_| ___|__,_|_|___||___/
[info]: Hercules 32-bit for Linux
[info]: Git revision (src): '47ff8ed7fa7603974a6f5e41b5290e5e24916317'
[info]: Git revision (scripts): '47ff8ed7fa7603974a6f5e41b5290e5e24916317'
[info]: OS version: 'Debian GNU/Linux 6.0.5 (squeeze) [i686]'
[info]: CPU: 'Intel® Xeon® CPU E3-1270 v3 @ 3.50GHz [1]'
[info]: Compiled with GCC v4.4.5
[info]: Compile Flags: -g -O2 -pipe -ffast-math -Wall -Wextra -Wno-sign-compare -march=i686 -Wno-unused-parameter -Wno-clobbered -Wempty-body -Wformat-security -Wno-format-nonliteral -Wno-switch -Wno-missing-field-initializers -fno-strict-aliasing -DMAXCONN=16384 -I../common -DHAS_TLS -DHAVE_SETRLIMIT -DHAVE_STRNLEN -I/usr/include -DHAVE_MONOTONIC_CLOCK
[info]: Server supports up to '1024' concurrent connections.
[Warning]: HPM:plugin_load: failed to load 'plugins/autopots.so' (error: plugins/autopots.so: cannot open shared object file: No such file or directory), skipping...
[status]: HPM: There are '1' plugins loaded, type 'plugins' to list them
make[1]: Entering directory `/home/xxx/trunk/src/plugins'
PLUGIN sample
PLUGIN db2sql
PLUGIN HPMHooking_char
PLUGIN HPMHooking_login
PLUGIN HPMHooking_map
PLUGIN auraset
CC autopots.c
autopots.c: In function 'autoatpots_timer':
autopots.c:79: warning: unused variable 'item'
autopots.c: In function 'atcommand_autopots':
autopots.c:133: warning: implicit declaration of function 'sscanf_s'
autopots.c: In function 'autoatpots_timer':
autopots.c:114: warning: array subscript is above array bounds
autopots.c:120: warning: array subscript is above array bounds
/tmp/ccxwrLU2.o: In function `atcommand_autosp':
/home/xxx/trunk/src/plugins/autopots.c:231: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:232: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:228: undefined reference to `sscanf_s'
/tmp/ccxwrLU2.o: In function `atcommand_autohp':
/home/xxx/trunk/src/plugins/autopots.c:189: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:190: undefined reference to `sscanf_s'
/tmp/ccxwrLU2.o:/home/xxx/trunk/src/plugins/autopots.c:186: more undefined references to `sscanf_s' follow
collect2: ld returned 1 exit status
make[1]: *** [../../plugins/autopots.so] Error 1
make[1]: Leaving directory `/home/xxx/trunk/src/plugins'
make: *** [plugins] Error 2
xxx@Nostalgic:~/trun
Any solutions ?
Cant reproduce. Make sure you are using version 4.1
Im using 4.1
now this
CC autopots.c
autopots.c: In function 'autoatpots_timer':
autopots.c:79: warning: unused variable 'item'
autopots.c: In function 'atcommand_autopots':
autopots.c:133: warning: implicit declaration of function 'sscanf_s'
autopots.c: In function 'autoatpots_timer':
autopots.c:114: warning: array subscript is above array bounds
autopots.c:120: warning: array subscript is above array bounds
/tmp/cccZYPnY.o: In function `atcommand_autosp':
/home/xxx/trunk/src/plugins/autopots.c:231: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:232: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:228: undefined reference to `sscanf_s'
/tmp/cccZYPnY.o: In function `atcommand_autohp':
/home/xxx/trunk/src/plugins/autopots.c:189: undefined reference to `sscanf_s'
/home/xxx/trunk/src/plugins/autopots.c:190: undefined reference to `sscanf_s'
/tmp/cccZYPnY.o:/home/xxx/trunk/src/plugins/autopots.c:186: more undefined references to `sscanf_s' follow
collect2: ld returned 1 exit status
make[1]: *** [../../plugins/autopots.so] Error 1
make[1]: Leaving directory `/home/xxx/trunk/src/plugins'
make: *** [plugins] Error 2
-
Can there be a queue system for this ? I mean change the registration type. Make it a waiting room and select randomly.
Example.
Two people are waiting outside. Then round 1 ends. Those two will be warped to each respected team.
-
Can someone modify the KVM and make it three rounds ? And also queue system would be appreciated.
-
Hello,
It will be awesome if you will share your solution here and please take time to read our Hercules Forum Rules.
Thank you. Did I violate any specific rule ?
invek,109,184,5 script Armor Enchant 430,{mes "Which Armor you want to Enchant?";mes "Make sure there is no Card/Rune in it.";next;switch(select("Non Slotted Armor.:Slotted Armor.:High Grade Armor.:Maybe next time.")) { case 1: setarray .EquipID[0],2307,2309,2314,2316,2321,2325,2327,2328,2330,2332,2334,2335,2341,2344,2346,2348,2350,2337,2386,2394,2395,2396; break; case 2: setarray .EquipID[0],2311,2318,2319,2320,2308,2310,2315,2317,2322,2324,2326,2331,2333,2336,2342,2345,2347,2349,2351; break; case 3: setarray .EquipID[0],2364,2365,2391,2374,2375,2376,2377,2378,2379,2380,2381,2382,2387,2388,2389,2390; break; case 4: mes "[Armor Enchanter]"; mes "Please come back when you have any interest in enchanting your armor."; close; }for( set .@i,0; .@i < getarraysize( .EquipID ); set .@i,.@i + 1 ){set .@EquipMenu$,.@EquipMenu$ + getitemname( .EquipID[.@i] )+( !getitemslots(.EquipID[.@i])?"":"["+getitemslots(.EquipID[.@i])+"]" )+":";}set .@Equip,select( .@EquipMenu$ ) - 1;if( !countitem( .EquipID[.@Equip] ) || countitem( 7227 ) < 5 ){mes "You didnt have this Equipment with you or you didnt have enough of 5 "+getitemname( 7227 );close;}mes "Equipment : ^FF0000"+getitemname( .EquipID[.@Equip] )+"^000000";switch( select( "Strength:Intelligent:Dexterity:Agility:Vitality:Luck" )){case 1: setarray .RuneID[0],4700,4701,4702; break;case 2: setarray .RuneID[0],4710,4711,4712; break;case 3: setarray .RuneID[0],4720,4721,4722; break;case 4: setarray .RuneID[0],4730,4731,4732; break;case 5: setarray .RuneID[0],4740,4741,4742; break;case 6: setarray .RuneID[0],4750,4751,4752; break;}for( set .@i,0; .@i < getarraysize( .RuneID ); set .@i,.@i + 1 ){set .@RuneMenu$,.@RuneMenu$ + getitemname( .RuneID[.@i] )+":";}set .@Rune,select( .@RuneMenu$ ) - 1;mes "Rune : ^FF0000"+getitemname( .RuneID[.@Rune] )+"^000000";mes "Cost : ^FF0000"+( 5 )+"^000000 TCG";if( countitem( .RuneID[.@Rune] ) < 1 ){mes "You didnt have the said orb.";close;}next;if( select("Confirm:Cancel") == 1 ){getitem2 .EquipID[.@Equip],1,1,0,0,0,0,0,.RuneID[.@Rune];delitem .EquipID[.@Equip],1;delitem 7227,5;delitem (.RuneID[.@Rune]),1;mes "Done.";mes "^0000FF"+getitemname( .EquipID[.@Equip] )+"^000000";mes "Enchanted with ^FF0000"+getitemname( .RuneID[.@Rune] )+"^000000";close;}}
Solved
-
Thank you for the clarification! Does the late click and effects delay apply also to 2012 clients?
Effects delay only (After 2012).
Reference (easy)
http://herc.ws/board/topic/8147-help-i-have-an-error-in-map-server/
Reference (details)
THANKS!
@autopots
in Plugin Support
Posted
No it's okay. I think I'll just pay malufett to fix it. Thank you!