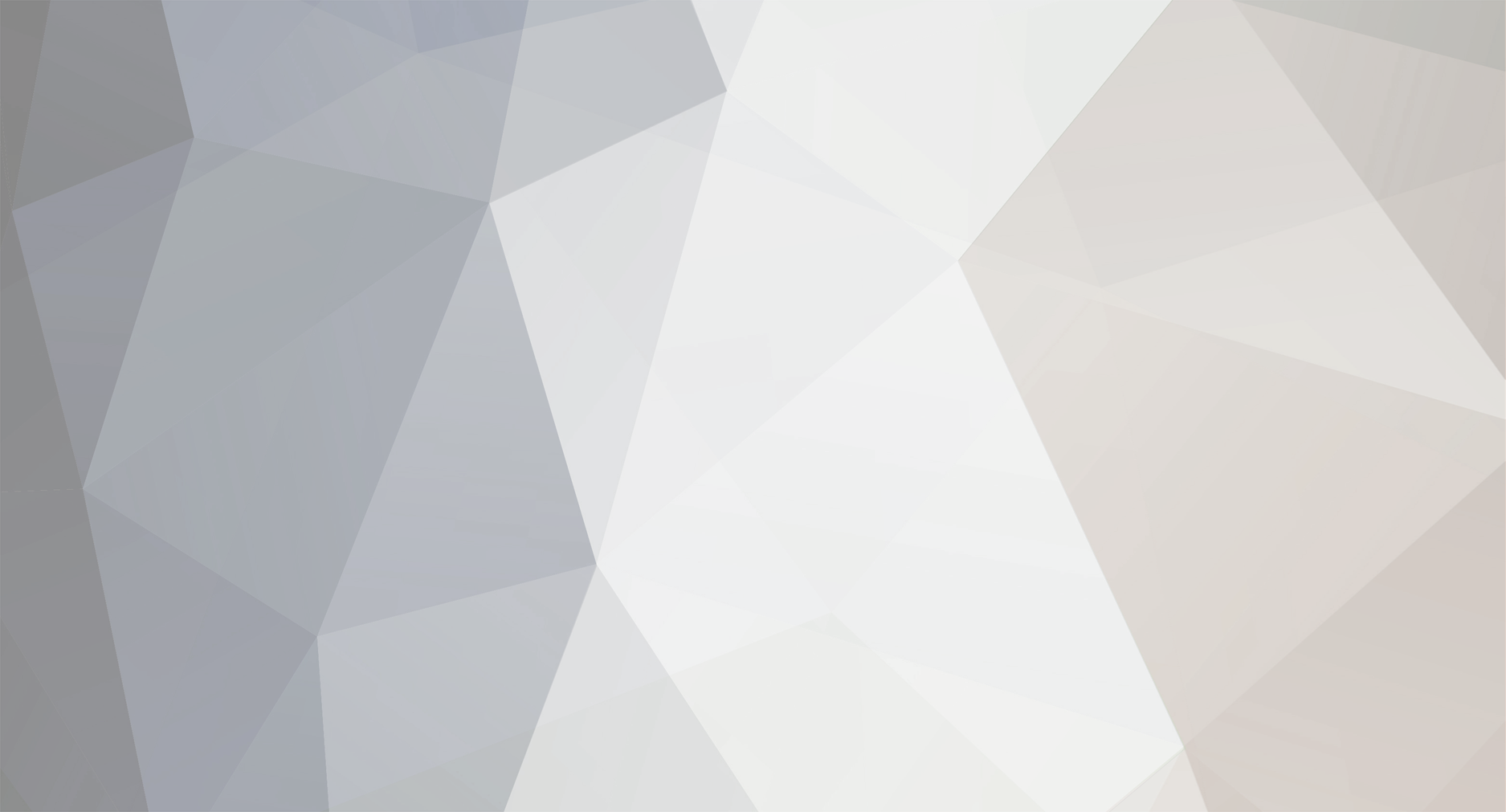
Playtester
Members-
Content Count
41 -
Joined
-
Last visited
-
Days Won
4
Content Type
Profiles
Forums
Downloads
Staff Applications
Calendar
Everything posted by Playtester
-
Um Michi, on Aegis ATK1 is base attack and ATK2 is attack variance. If you convert it you need to do: ATK1 = ATK1 ATK2 = ATK1+ATK2 Unless you changed the code to interpret the values differently, but in that case you should also update mobinfo to display ATK1+ATK2 as the second number instead of just ATK2, otherwise it's hard to read. Players want to see min damage and max damage and not the variance.
-
Well in that case just find: //Aegis accuracy if(rate > 0 && rate%10 != 0) rate += (10 - rate%10);}And then add: //Aegis accuracy if(rate > 0 && rate%10 != 0) rate += (10 - rate%10); //Immunity at 300 Luk if(st->luk > 299) rate = 0;}
-
Well 300 is ugly number, because 10000 can't be divided by it. You could for example make each Luk give 33 scdef for the corresponding status change. Maybe I can give other suggestions, but I need to know what your core issue is. Do you think that Luk gives too much resistance right now or too little? Do you only care about a specific status change or do you want all status changes only depend on Luk? Should there be other stats affecting the resistances? How should different resistances stack? Should Luk reduce the chance gradually or should it be 299 Luk 100% chance, 300 Luk 0% chance? As you can see there are a lot different possible implementations.
-
Deprecated, as the official status resistance formulas have been added and they are very complex and different for every single status change so a general option for luk wasn't viable anymore. The normal status DEF rate option now applies to all stats that are involved in the resistance: // Adjustment for the natural rate of resistance from status changes.// If 50, status defense is halved, and you need twice as much stats to block// them (eg: 200 vit to completely block stun)pc_status_def_rate: 100mob_status_def_rate: 100You can also manually change the individual status resistances fairly easily via the code.Just look at status.c and search for "int status_get_sc_def". Just understand sc_def as percentual def and sc_def2 as linear def (kind of like armor DEF / vit DEF in pre-renewal). tick_def and tick_def2 is for duration respectively. You don't need to set tick_def specifically as by default it uses sc_def.
-
How to adjust STAT requirements for Status effect?
Playtester replied to Hadeszeus's question in Source Support
If you just want more stats required in general for all status changes and not change the stats, then there is also a config for it in status.conf. :-) https://raw.githubusercontent.com/HerculesWS/Hercules/master/conf/battle/status.conf // Adjustment for the natural rate of resistance from status changes.// If 50, status defense is halved, and you need twice as much stats to block// them (eg: 200 vit to completely block stun)pc_status_def_rate: 100mob_status_def_rate: 100 -
Summoned monster sprite size // monster having HP-Bar
Playtester replied to Thuan's question in Client-Side Support
Are you using the @monster command? Because that looks like you used @monstersmall. See groups.conf: -
Tough one, Stormgust right now uses all the default settings for ground skill. That's why you couldn't find it. It's actually pretty wrong and I totally would want to fix it for pre-renewal if I could. ^^; But anyway, try it here: int skill_unit_onplace_timer(struct skill_unit *src, struct block_list *bl, int64 tick) { https://raw.githubusercontent.com/HerculesWS/Hercules/master/src/map/skill.c I think that's the function that gets called each ground hit interval. Check out the part for FIREWALL for example, where it does multiple hits during one interval. That's kind of similar to what you want. To do it only every second hit you will need to check the sg_counter variable saved in the target struct (tsc I think).
- 2 replies
-
- Storm Gust
- WZ_STORMGUST
-
(and 2 more)
Tagged with:
-
ad.damage will contain the amount that is healed (for heal skills)
-
1 - Not sure what exactly you mean but if you want a skill to have multiple hits you need to set the number of hits too: // 09 Number of hits (when positive, damage is increased by hits, // negative values just show number of hits without increasing total damage) 2 - You would need to do that in the code. I guess one place you can put that is battle_calc_magic_attack in battle.c: struct Damage battle_calc_magic_attack(struct block_list *src,struct block_list *target,uint16 skill_id,uint16 skill_lv,int mflag)Add a "case" for AB_CHEAL and then set hp to a percent of maxhp of tstatus, like:case AB_CHEAL: ad.damage = (tstatus->max_hp * skill_lv) / 10; break;Make sure you set up the skill as magic skill or else it won't reach that function code. I couldn't find the skill AB_CHEAL in the code at all, so I just assumed you define it similar to AB_HIGHNESSHEAL and the likes. PS: If you mean current HP instead of max HP just use tstatus->hp instead.
-
Changing the type of stat needed itself no. If you just want everything to require 4 times higher stats then you can use the solution arisgamers posted:
-
Not quite. What you want is that: case SC_FREEZE: sc_def = st->luk*25; tick_def = 0; //No duration reduction break;And:case SC_STUN: sc_def = st->vit*50; tick_def = 0; //No duration reduction break;You can remove all the rest of the code for those status changes.
-
Depends a bit on what exactly you want. Status change resistances are quite complex. They work a bit like normal def as in there is a hard def (percentual reduction) and a soft def (linear reduction). I wrote a guide about them over on RMS: http://forum.ratemyserver.net/guides/guide-official-status-resistance-formulas-%28pre-renewal%29/ So, do you just want all status changes to depend only on LUK? Or get reduced by other stats? What should affect the chance? What should affect the duration? Etc. You can find the relevant source code for the status changes in the link that csnv posted. Basically if you want to change the basic stats a status change uses, you need to change it for each status change individually, see explanations in the comments of the source code about how sc_def, sc_def2, tick_def and tick_def2 work (10000 sc_def = 100% resistance). It's actually pretty straight forward. For example if you want each LUK to reduce chance and duration of STUN by 0.25% that code would look like this: case SC_STUN: sc_def = st->luk*25; break;(Note: You don't need to set tick_def, it automatically uses sc_def if you don't set it.) Or if you set the sc_def config to 25 instead of 100 then you would write: case SC_STUN: sc_def = st->luk*100; break;The config option is applied at the end: if (battle_config.pc_sc_def_rate != 100) { sc_def = sc_def*battle_config.pc_sc_def_rate/100; sc_def2 = sc_def2*battle_config.pc_sc_def_rate/100; }If you leave the source code as it is and just set the config option to 25, then the status changes simply need 4 times higher stats than they usually need. As LUK has a very high effect on the linear reduction, you will probably be immune to any status change much earlier. Hope it helps. If you explain in detail why you want to change it and how exactly it should work, I could probably help more.
-
case UNT_EVILLAND: //Will heal demon and undead element monsters, but not players. if ((bl->type == BL_PC) || (!battle->check_undead(tstatus->race, tstatus->def_ele) && tstatus->race!=RC_DEMON)) { //Damage enemies if(battle->check_target(&src->bl,bl,BCT_ENEMY)>0) skill->attack(BF_MISC, ss, &src->bl, bl, sg->skill_id, sg->skill_lv, tick, 0); } else { int heal = skill->calc_heal(ss,bl,sg->skill_id,sg->skill_lv,true); if (tstatus->hp >= tstatus->max_hp) break; if (status->isimmune(bl)) heal = 0; clif->skill_nodamage(&src->bl, bl, AL_HEAL, heal, 1); status->heal(bl, heal, 0, 0); } break;Was intentionally coded so that players are never healed by it (plus shadow element is never healed by it anyway, only demon race and undead element). Hmm... skill->attack(BF_MISC, ss, &src->bl, bl, sg->skill_id, sg->skill_lv, tick, 0); Maybe element doesn't work because it's defined as MISC damage here? In battle_calc_misc_attack: s_ele = skill->get_ele(skill_id, skill_lv); if (s_ele < 0 && s_ele != -3) //Attack that takes weapon's element for misc attacks? Make it neutral [Skotlex] s_ele = ELE_NEUTRAL; else if (s_ele == -3) //Use random element s_ele = rnd()%ELE_MAX;Don't see how it goes through there without setting s_ele to 7. *rubs head*
-
Hmm... Evil Land is correctly defined as Dark Element. skill_db.txt attr_fix.txt Dark 1 takes exactly 0% damage from Dark.Should it really heal? If you checked those two files and those value are in there, the problem must be somewhere in the source code I guess. Let's see...
-
//Call skill->trap_splash for all monster in splash area mobCount times map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag|BL_SKILL|~BCT_SELF, &src->bl,tick); If you want all hits to be displayed, you need to send a different tick for each hit like for example: //Call skill->trap_splash for all monster in splash area mobCount times map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag|BL_SKILL|~BCT_SELF, &src->bl,tick+(50*i)); That would put a 50ms delay between each hit. Hope it helps! Also: I probably should mention this: When you test, don't just let mobs run on the trap. Spawn several immobile mobs on a 3x3 area, put Claymore trap next to them, then use Arrow Shower to knock the trap in the middle of the mobs. If it does multi-hit then, it works just like on official servers (before the 2011 fix).
-
Yeah, the solution Malufett posted looks like it will just make it hit for a fixed amount of time in regular intervals based on level. Basically like Sanctuary works (but with damage instead of heal). You get the trap back because the unit is never set to UNT_USED_TRAPS. Also if you want the official implementation of the skill, then it should only count the mobs on a 3x3 area around the trap (trigger area) and not all mobs in the splash area (5x5). And it should also work for other traps actually (though I guess Claymore Trap is the only popular trap to be used for this). You have to use something similar to Ind's original suggestion. I would even do it so it doesn't show multi hit... only causes lag anyway. But matter of taste I guess. Current code is also a bit differerent now and looks like this: case UNT_CLAYMORETRAP: if( sg->unit_id == UNT_FIRINGTRAP || sg->unit_id == UNT_ICEBOUNDTRAP || sg->unit_id == UNT_CLAYMORETRAP ) map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag|BL_SKILL|~BCT_SELF, &src->bl,tick); else map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag, &src->bl,tick); if (sg->unit_id != UNT_FIREPILLAR_ACTIVE) clif->changetraplook(&src->bl, sg->unit_id==UNT_LANDMINE?UNT_FIREPILLAR_ACTIVE:UNT_USED_TRAPS); sg->limit=DIFF_TICK32(tick,sg->tick)+1500 + (sg->unit_id== UNT_CLUSTERBOMB || sg->unit_id== UNT_ICEBOUNDTRAP?1000:0);// Cluster Bomb/Icebound has 1s to disappear once activated. sg->unit_id = UNT_USED_TRAPS; //Changed ID so it does not invoke a for each in area again. break;The idea to not set it to UNT_USED_TRAPS is nice, but it doesn't work because you will also need to remember which monster already triggered the trap in this case (aka each monster can only trigger it once). Also there needs to be a final condition that sets it to UNT_USED_TRAPS a few milliseconds after first activation.Kind of difficult to code but not impossible. Ind's solution is easier and should always work but I'd to it like on officials and only count 3x3 activation area monsters: Y'know like this: case UNT_CLAYMORETRAP:if( sg->unit_id == UNT_CLAYMORETRAP ) { //Count total number of mobs in 3x3 range around trap int mobcount = map->foreachinrange(skill->area_sub, &src->bl, 1, BL_CHAR, &src->bl, sg->skill_id, sg->skill_lv, tick, BCT_ENEMY, skill->area_sub_count); if( mobcount <= 0 ) mobcount = 1; //Should never happen because something must have triggered the trap, but to make sure for( int i = 0; i < mobCount; i++ ) { //Call skill->trap_splash for all monster in splash area mobCount times map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag|BL_SKILL|~BCT_SELF, &src->bl,tick); }} else if( sg->unit_id == UNT_FIRINGTRAP || sg->unit_id == UNT_ICEBOUNDTRAP ) map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag|BL_SKILL|~BCT_SELF, &src->bl,tick);else map->foreachinrange(skill->trap_splash,&src->bl, skill->get_splash(sg->skill_id, sg->skill_lv), sg->bl_flag, &src->bl,tick);if (sg->unit_id != UNT_FIREPILLAR_ACTIVE) clif->changetraplook(&src->bl, sg->unit_id==UNT_LANDMINE?UNT_FIREPILLAR_ACTIVE:UNT_USED_TRAPS);sg->limit=DIFF_TICK32(tick,sg->tick)+1500 + (sg->unit_id== UNT_CLUSTERBOMB || sg->unit_id== UNT_ICEBOUNDTRAP?1000:0);// Cluster Bomb/Icebound has 1s to disappear once activated.sg->unit_id = UNT_USED_TRAPS; //Changed ID so it does not invoke a for each in area again.break;